Mastering API Integration in React Native
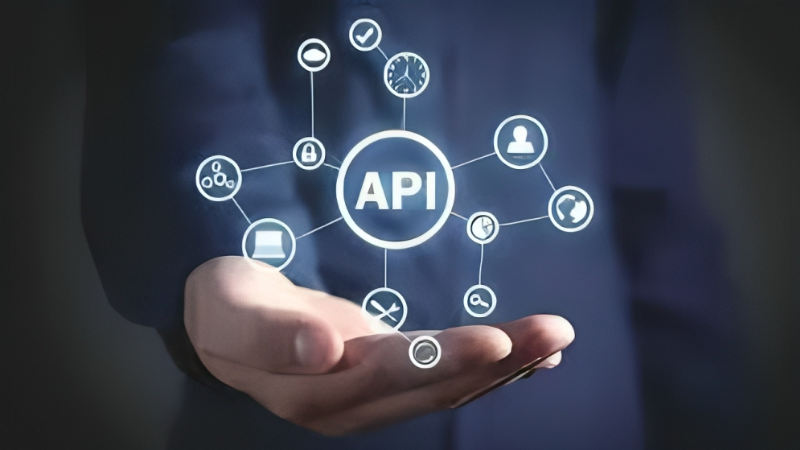
Introduction: React Native, the beloved framework for creating cross-platform mobile applications using JavaScript and React, is powerful. But what makes it even more potent? APIs. These little gateways to the vast world of data and services are what give modern mobile apps their edge. In this comprehensive guide, tailored for React Native course students, we'll unravel the art of API integration in React Native.
Understanding the Role of APIs in React Native
Before we dive into the technicalities, let's clarify what APIs are all about in React Native. APIs, or Application Programming Interfaces, are the essential connectors that enable your app to communicate with external services, be it servers, databases, or other applications. In React Native, APIs serve as the lifelines for accessing data and features from external sources.
Making API Calls: The Foundation
Fetching data from APIs is the bread and butter of React Native app development. To start, we use methods like fetch()
or explore third-party libraries such as Axios or Fetch. These tools allow us to send HTTP requests to API endpoints and retrieve responses in various formats, often JSON or XML.
Here's a fundamental example of fetching data from an API endpoint in React Native:
javascriptCopy codefetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
// Handle the retrieved data here
})
.catch(error => {
// Handle errors gracefully
console.error(error);
});
Selecting the Right API
Choosing the correct API is a critical step. Consider factors like data quality, reliability, and cost when making your selection. Also, ensure that the API offers comprehensive documentation and endpoints that align with your app's requirements.
Handling Slow Network Connections
Real-world network conditions vary. To create a user-friendly experience, you should account for slow network connections. React Native offers libraries like NetInfo to detect the network type and adjust your app's behavior accordingly. Display loading indicators or optimize data fetching to cater to different network speeds.
Inspecting API Calls
Debugging is an integral part of development. Tools like console.log()
, browser dev tools' network tab, or third-party tools like Postman help inspect API calls. This process can unveil issues with request headers, response data, or unexpected behaviors.
Error Handling: Keeping Your App Robust
Effective error handling is non-negotiable. In React Native, you have multiple options:
Error Boundaries
React components known as Error Boundaries can capture JavaScript errors in their child component tree. They provide a safety net, allowing you to display error messages gracefully instead of crashing the entire app.
javascriptCopy codec
lass ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false }; } static getDerivedStateFromError(error) { return { hasError: true }; } render() { if (this.state.hasError) { return <h1>Something went wrong.</h1>; } return this.props.children; } }
Requesting Interceptors
Interceptors are your allies in modifying outgoing requests or incoming responses. Use them to add headers, log activities, or transform data. Properly handling interceptors can streamline your error management and response processing.
Explicit Error Handling
Sometimes, it's best to handle errors explicitly. This approach involves checking for errors within your code using conditional statements or try-catch blocks. When an error occurs during an API call, you can present relevant error messages to the user, offering guidance on resolving the issue.
javascriptCopy codeconst getData = async () => { try { const response = await fetch('https://my-api.com/data'); const data = await response.json();
return data; } catch (error) { console.error(error); // Handle error here, e.g., show an error message to the user } }
Simulating Slow Network Connections
Simulating slow network connections is a smart testing practice. By adding delays to your API requests using setTimeout()
or combining it with the NetInfo library, you can evaluate how your app performs under adverse network conditions. This helps identify potential issues related to timeouts, error handling, and user experience.
Conclusion: Empowering React Native with API Integration
APIs are the lifeblood of modern mobile applications. They empower React Native apps with access to data and services beyond their local boundaries. The fetch() method and third-party libraries like Axios enable you to harness the potential of APIs effectively. Combine this with robust error handling strategies, and your React Native app will stand strong even in the face of network challenges.
So, fellow React Native enthusiasts, embrace the power of APIs and create dynamic, data-driven mobile apps that captivate your users. Happy coding!